New Keyword in Java
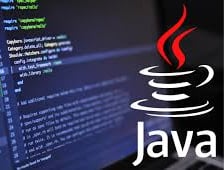
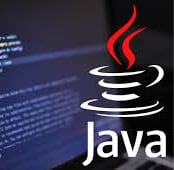
"The new keyword is a cornerstone of object-oriented programming in Java, enabling developers to create and initialize objects dynamically. A solid grasp of its functionality is essential for effective Java development."
What is the new Keyword in Java?
The new keyword in Java is used to instantiate new objects. When you create an object using new, it allocates memory for the object and returns a reference to that memory. This process involves calling the constructor of the class, which initializes the new object.
Syntax:
ClassName objectName = new ClassName(parameters);
Example:
Creating an object of the 'Car' class Car myCar = new Car();
In this example, Car() is the constructor of the Car class. The new keyword allocates memory for the myCar object and initializes it using the Car constructor.
Uses of the new Keyword
Object Creation: The primary use of the new keyword is to create instances of classes.
Dog myDog = new Dog();
Array Creation: The new keyword is also used to create arrays.
int[] numbers = new int[5];
Anonymous Object Creation: Sometimes, new is used to create anonymous objects, often in scenarios like passing an object as an argument to a method.
someMethod(new SomeClass());
How the new Keyword Works
When the new keyword is used, the following steps occur:
Memory Allocation: Memory is allocated from the heap for the new object.
Constructor Call: The constructor of the class is invoked to initialize the object.
Reference Assignment: A reference to the newly created object is returned and can be assigned to a variable.
It's important to note that if a constructor is not explicitly defined in a class, Java provides a default no-argument constructor.
Best Practices
Constructor Initialization: Always ensure that your constructors properly initialize object fields to maintain data integrity.
Avoid Memory Leaks: Be cautious of creating objects unnecessarily, as it can lead to memory leaks. Properly manage object references and utilize garbage collection.
Use of Anonymous Objects: While anonymous objects can be useful, ensure they are used judiciously to maintain code readability and manageability.